export JAVA_HOME=/Library/Java/Home
export PATH="$PATH:$JAVA_HOME/bin"
2 Getting Started
Version: 7.0.0-SNAPSHOT
Table of Contents
2 Getting Started
2.1 Installation Requirements
Before installing Grails you will need a Java Development Kit (JDK) installed with the minimum version denoted in the table below. Download the appropriate JDK for your operating system, run the installer, and then set up an environment variable called JAVA_HOME
pointing to the location of this installation.
We recommend Liberica JDK 17 LTS based on Spring’s JDK recommendation.
Grails version | JDK version (minimum) |
---|---|
7 |
17 |
6 |
11 |
5 |
8 |
To automate the installation of Grails we recommend SDKMAN which greatly simplifies installing and managing multiple Grails versions.
On some platforms (for example macOS) the Java installation is automatically detected. However in many cases you will want to manually configure the location of Java. For example, if you’re using bash or another variant of the Bourne Shell:
On Windows you would have to configure these environment variables in My Computer/Advanced/Environment Variables
|
2.2 Downloading and Installing
There are many ways to create a Grails application. The best way is to use either start.grails.org or use the Grails CLI via SDKMAN. SDKMAN greatly simplifies installing and managing multiple Grails versions.
Types of command-line interface (CLI)
Historically, Grails had one CLI - grails shell
, until grails forge
was introduced in Grails 6.
Grails Shell CLI
The historical grails shell
CLI has been a core part of the Grails Framework since its inception, providing command-line tools for project creation, code generation, and application management.
Nearly all historical references to the grails
or grailsw
commands pertain to grails shell
functionality.
The grails shell
CLI relies on Profiles, consisting of skeleton directories and YAML-based configurations for application generation.
However, this approach is less flexible than the feature-rich application generation capabilities of grails forge
.
The Grails Wrapper is a lightweight script (grailsw
) and the accompanying JAR file is designed to bootstrap and load the CLI within a Grails Application.
The grails shell
CLI provides most of the same application generation functionality provided by grails forge
.
Importantly, it can also run scripts, such as run-app
that call Gradle and/or your Grails Application.
Most Grails plugins still include Grails Scripts that execute within grails shell
CLI and have not yet been migrated to Gradle tasks.
IntelliJ IDEA’s Grails plugin depends on grails shell
for code generation and Grails command execution.
Extending application generation in the grails shell CLI is straightforward: developers can create a Custom Profile and reference it as a Maven dependency, which is generally simpler than customizing grails forge
.
Restoration of Grails Shell CLI
In Grails 6.0.0, grails shell
CLI was removed and replaced with grails forge
CLI.
However, in Grails 6.2.1, the grails shell CLI, along with Grails Profiles and the Wrapper were restored, empowering users to choose their preferred CLI and reclaiming functionalities that had not yet been fully transitioned to the grails forge
CLI combined with Gradle tasks.
Grails Shell CLI and Gradle
Certain grails shell
CLI commands overlap with Gradle tasks.
For Gradle these use a variety of Gradle tasks provided by Gradle, Spring Boot, Grails or a Grails Plugin.
Partial list, for illustration purposes:
grails shell CLI | Gradle | Integration |
---|---|---|
|
|
run-app is a Grails Script from the Base Grails Profile commands directory and ultimately calls the Gradle bootRun task |
|
|
run-app is a Grails Script from the Base Grails Profile commands directory and ultimately calls the Gradle bootRun task |
|
|
package is a YAML file from the Base Grails Profile commands directory which is converted by YamlCommandFactory, when Grails CLI starts, into a Grails Command which ultimately calls the Gradle assemble task |
|
|
None. The Grails Script GenerateAll.groovy and the Grails Command GenerateAllCommand.groovy both come from the Scaffolding Plugin and have duplicate functionality |
|
|
None. Separate script and command in Database Migration Plugin |
|
|
None. Separate script and command in Database Migration Plugin |
|
|
None. The Grails Script s2-quickstart.groovy and the Grails Command S2QuickstartCommand.groovy both come from the Grails Spring Security Core Plugin and have duplicate functionality |
Additional details on these commands are covered in other sections of Grails documentation.
Grails Forge CLI
In Grails 6, grails forge
was introduced to replace the application generation features of grails shell
CLI, while forcing the transition of all other historical CLI functionality directly to Gradle tasks.
This new CLI offers a superior architecture for application generation, particularly when incorporating multiple features, and starts and generates applications faster than the grails shell
CLI.
grails forge
also powers start.grails.org and the "New Grails Project" feature in JetBrains' IntelliJ IDEA via the Grails Plugin.
Unfortunately, in Grails 6, but corrected for 7, the grails forge CLI was invoked using the historical grails
command, which confused users due to its markedly different feature set and architecture.
The grails forge
CLI is dedicated solely to initial application generation and the subsequent creation of components such as domains, controllers, services, interceptors, and tag libraries, which is all it can do.
This CLI is entirely offline with precompiled templates and does not run or use Gradle or your Grails Application.
With the grails forge
CLI, the goal was to transition all other historical capabilities provided by the grails shell
CLI to Gradle tasks.
Although some advancements have been made in this transition, substantial work is still outstanding.
The grails forge
can be extended by forking the repository and hosting your own Grails Application Forge internally, which is generally more complex than extending grails shell
with a custom profile.
Both CLIs exist in Grails 7
For the reasons listed above, Grails 7 now includes both CLIs and a combined delegating CLI that can invoke either CLI. grails-shell
, grails-profiles
, grails-wrapper
and grails-forge
projects are now all part of the grails-core project and are released together.
Grails 7 adds the following commands to any Grails install:
-
grails-forge-cli
- the forge cli that was shipped asgrails
initially in Grails 6. This CLI has both interactive completion & bash autocompletion. Long term, we intend to fold the legacy shell functions into it - including the ability to create custom commands & supporting a custom layout. -
grails-shell-cli
- the legacy shell cli that IntelliJ uses. This CLI has only interactive completion. Historically, this shell has not had autocompletion. -
grails
- a new, delegating shell application that will call eithergrails-shell-cli
orgrails-forge-cli
. By default,./grails
usesgrails-shell-cli
for backwards compatibility. To usegrails-forge-cli
, pass the-t
or--type
argument:$ ./grails -t forge
Install with SDKMAN
To install the latest version of Grails using SDKMAN, run this on your terminal:
$ sdk install grails
You can also specify a version
$ sdk install grails 7.0.0-SNAPSHOT
You can find more information about SDKMAN usage on the SDKMAN Docs.
After installing, all three CLI commands: grails
, grails-shell-cli
, and grails-forge-cli
will be available.
Manual installation
For manual installation follow these steps:
-
Download a binary distribution of Grails and extract the resulting zip file to a location of your choice. Then add the bin directory to your system path.
Unix/Linux
-
This can be done by adding
export PATH="$PATH:/path/to/grails/bin"
to your profile
Windows
-
Copy the path to the bin directory inside the grails folder you have downloaded, for example,
C:\path_to_grails\bin
-
Go to Environment Variables, you can typically search or run the command below, the type env and then Enter
Start + R
-
Edit the Path variable on User Variables / System Variables depending on your choice.
-
Paste the copied path in the Path Variable.
If Grails is working correctly you should now be able to type grails --version
in the terminal window and see output similar to this:
Grails Version: 7.0.0-SNAPSHOT
Grails Wrapper
The original CLI binaries can be quite large.
When installing via SDKMAN or via a download, we include a single shadowJar that contains both shells and the associated scripts to invoke them.
However, when a project is generated, we also include a wrapper grailsw
that can be checked into source control.
This wrapper will download the CLI’s to ~/.grails/wrapper/myGrailsVersion
and invoke them when calling grailsw
.
It is meant to be a light weight option if SDKMAN is not available.
2.3 Creating an Application
To create a Grails application you first need to familiarize yourself with the usage of the grails
command which is used in the following manner:
$ grails <<command name>>
Run create-app to create an application:
The create-app command is supported by both the Grails Shell CLI and the Grails Forge CLI.
|
Grails Shell CLI
$ grails create-app myapp
Grails Forge CLI
$ grails -t forge create-app myapp
This will create a new directory inside the current one that contains the project. Navigate to this directory in your console:
$ cd myapp
2.4 Creating a Simple Web Application with Grails
Step 1: Create a New Project
Open your command prompt or terminal.
Navigate to the directory where you want to create your Grails project:
$ cd your_project_directory
Create a new Grails project with the following command:
$ grails -t forge create-app myapp
You can omit -t forge , to generate the application with Grails Shell CLI and profiles
|
Step 2: Access the Project Directory
Change into the "myapp" directory, which you just created:
$ cd myapp
Step 3: Start Grails Interactive Console
Start the Grails Forge CLI interactive console by running the "grails" command:
$ grails -t forge
Step 4: Create a Controller
In the Grails interactive console, you can use auto-completion to create a controller. Type the following command to create a controller named "greeting":
grails> create-controller greeting
This command will generate a new controller named "GreetingController.groovy" within the grails-app/controllers/myapp directory. You might wonder why there is an additional "myapp" directory. This structure aligns with conventions commonly used in Java development, where classes are organized into packages. Grails automatically includes the application name as part of the package structure. If you do not specify a package, Grails defaults to using the application name.
For more detailed information on creating controllers, you can refer to the documentation on the create-controller page.
Step 5: Edit the Controller
Open the "GreetingController.groovy" file located in the "grails-app/controllers/myapp" directory in a text editor.
Add the following code to the "GreetingController.groovy" file:
package myapp
class GreetingController {
def index() {
render "Hello, Congratulations for your first Grails application!"
}
}
The action is simply a method. In this particular case, it calls a special method provided by Grails to render the page.
Step 6: Run the Application
Grails framework now relies on Gradle tasks for running the application. To start the application, use the following Gradle bootRun
command:
$ ./gradlew bootRun
Your application will be hosted on port 8080 by default. You can access it in your web browser at:
Now, it’s important to know that the welcome page is determined by the following URL mapping:
class UrlMappings {
static mappings = {
"/$controller/$action?/$id?(.$format)?"{
constraints {
// apply constraints here
}
}
"/"(view:"/index")
"500"(view:'/error')
"404"(view:'/notFound')
}
}
This mapping specifies that the root URL ("/") should display the "index.gsp" view, which is located at "grails-app/views/index.gsp." This "index.gsp" file serves as your welcome or landing page. The other entries in the mapping handle error pages for HTTP status codes 500 and 404.
Grails URL Convention Based on Controller and Action Name
Grails follows a URL convention that relies on the names of controllers and their actions. This convention simplifies the creation and access of various pages or functionalities within your web application.
In the provided code example:
package myapp
class GreetingController {
def index() {
render "Hello, Congratulations for your first Grails application!"
}
}
-
The
GreetingController
class represents a controller in Grails. -
Inside the controller, there’s an
index
action defined as a method. In Grails, actions are essentially methods within a controller that handle specific tasks or respond to user requests.
Now, let’s understand how the Grails URL convention works based on this controller and action:
-
Controller Name in URL:
-
The controller name, in this case, "GreetingController," is used in the URL. However, the convention capitalizes the first letter of the controller name and removes the "Controller" suffix. So, "GreetingController" becomes "greeting" in the URL.
-
-
Action Name in URL:
-
By default, if you don’t specify an action in the URL, Grails assumes the "index" action. So, in this example, accessing the URL
/greeting
-
See the end of the controllers and actions section of the user guide to find out more on default actions.
Optional: Set a Context Path
If you want to set a context path for your application, create a configuration property in the "grails-app/conf/application.yml" file:
server:
servlet:
context-path: /myapp
With this configuration, the application will be available at: http://localhost:8080/myapp/
Alternatively, you can also set the context path via the command line:
grails> run-app -Dgrails.server.servlet.context-path=/helloworld
Alternatively, you can set the context path from the command line when using Gradle to run a Grails application. Here’s how you can do it:
$ ./gradlew bootRun -Dgrails.server.servlet.context-path=/your-context-path
Replace /your-context-path
with the desired context path for your Grails application. This command sets the context path directly via the -Dgrails.server.servlet.context-path
system property.
For example, if you want your application to be available at "http://localhost:8080/myapp," you can use the following command:
$ ./gradlew bootRun -Dgrails.server.servlet.context-path=/myapp
This allows you to configure the context path without modifying the application’s configuration files, making it a flexible and convenient option when running your Grails application with Gradle.
Optional: Change Server Port
If port 8080 is already in use, you can start the server on a different port using the grails.server.port
system-property:
$ ./gradlew bootRun -Dgrails.server.port=9090
Replace "9090" with your preferred port.
Note for Windows Users
It may also be necessary to enclose the system properties in quotes on Windows:
./gradlew bootRun "-Dgrails.server.port=9090"
Conclusion
Your Grails application will now display a "Hello, Congratulations on your first Grails application!" message when you access it in your web browser.
Remember, you can create multiple controllers and actions to build more complex web applications with Grails. Each action corresponds to a different page accessible through unique URLs based on the controller and action names.
2.5 Using Interactive Mode
Since 3.0, Grails has an interactive mode which makes command execution faster since the JVM doesn’t have to be restarted for each command. To use interactive mode simply type 'grails' from the root of any projects and use TAB completion to get a list of available commands. See the screenshot below for an example:
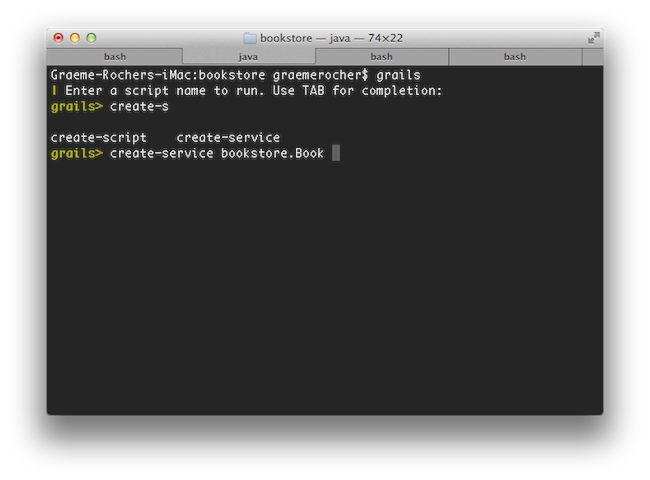
As of Grails 7, both the Grails Shell CLI and the Forge CLI are available. Interactive mode is part of the Grails Shell CLI and is accessed by typing grails without additional arguments. Grails Forge CLI is accessed as grails -t forge .
|
For more information on the capabilities of interactive mode refer to the section on Interactive Mode in the user guide.
The Grails Shell Command-line Interface (CLI) offers an interactive mode, which you can access by entering "grails" in your Terminal application or Linux Command Line.
Once you’re in the command-line interface, you can enhance your efficiency by utilizing the TAB key for auto-completion. For instance:
grails> create
create-app create-plugin create-webapp
create-controller create-restapi
create-domain-class create-web-plugin
This interactive mode provides a convenient way to explore available Grails commands and options, making your Grails development workflow more efficient and user-friendly.
For more information on the capabilities of interactive mode, refer to the section on Interactive Mode in the user guide.
2.6 Getting Set Up in an IDE
Because Grails is built upon the Spring Framework (Spring Boot), the Gradle build tool, and the Groovy programming language, it is possible to develop Grails application using most popular JVM Integrated Development Environments (IDEs). Some IDEs offer more specialized support for Grails, while others may offer basic support for managing dependencies/plugins, running Gradle tasks, code-completion and syntax highlighting.
1. IntelliJ IDEA
IntelliJ IDEA is a widely used IDE for Grails development. It offers comprehensive support for Groovy and Grails, including features like code-completion, intelligent code analysis, and seamless integration with Grails artefacts.
The new Apache Grails Maven Coordinates are supported in IntelliJ IDEA 2025.2 and later releases via the Grails Plugin.
IntelliJ IDEA also provides powerful database tools that work with Grails' GORM (Grails Object Relational Mapping) seamlessly. It offers both a Community (free) and Ultimate (paid) edition, with the latter offering more advanced Grails support via the regularly updated Grails Plugin, including an embedded version of the Grails Forge, and view resolution for both GSPs and JSON views.
For larger projects, it may be beneficial to adjust memory settings, based on available system RAM, by increasing -Xmx (maximum Java heap size) and -XX:ReservedCodeCacheSize (JIT compiler maximum code cache size). Edit these settings under Help→Custom VM Options , by adding one setting per line. Example for a small project: -Xmx2048m -XX:ReserveCodeCacheSize=128m and for a large project: -Xmx6144m -XX:ReservedCodeCacheSize=512m
|
2. Visual Studio Code (VS Code)
Visual Studio Code is a lightweight, open-source code editor developed by Microsoft. While it’s not a full-fledged IDE, it offers powerful extensions for Grails and Groovy development. You can install extensions like code-groovy and Grails for VS Code to enhance your Grails developer experience.
VS Code provides features such as syntax highlighting, code navigation, and integrated terminal support. It’s a great choice for developers who prefer a lightweight and customizable development environment.
3. STS (Spring Tool Suite)
The Spring Tool Suite (STS) is set of IDE tools designed for Spring Framework development, with versions based on both VS Code and Eclipse. This section focuses on the Eclipse version.
STS can work as an effective Grails developer platform when used with the Groovy Development Tools plugin (which can be installed using the Eclipse Marketplace). STS does not offer specific support for Grails artefacts or GSP views.
4. Netbeans
Apache Netbeans does not offer specific support for Grails, but it will import Grails applications as Gradle projects and provides reasonable editing support for Groovy and GSP views.
5. TextMate, VIM, and More
There are several excellent text editors that work nicely with Groovy and Grails. Here are some references:
-
A bundle is available for Groovy / Grails support in Textmate.
-
A plugin can be installed via Sublime Package Control for the Sublime Text Editor.
-
The emacs-grails extension offers basic support for Grails development in Emacs.
-
See this post for some helpful tips on how to set up VIM as your Grails editor of choice.
These text editors, along with the provided extensions and configurations, can enhance your Groovy and Grails development experience, offering flexibility and customization to meet your coding preferences.
2.7 Grails Directory Structure and Convention over Configuration
Grails adopts the "convention over configuration" approach to configure itself. In this approach, the name and location of files are used instead of explicit configuration. Therefore, it’s essential to become familiar with the directory structure provided by Grails. Here’s a breakdown of the key directories and links to relevant sections:
-
grails-app
- Top-Level Directory for Groovy Sources-
conf
- Configuration Sources -
controllers
- Web Controllers - Responsible for the "C" in MVC (Model-View-Controller). -
domain
- Application Domain - Represents the "M" in MVC. -
i18n
- Supports Internationalization (i18n). -
services
- The Service Layer. -
taglib
- Tag Libraries. -
utils
- Houses Grails-specific utilities. -
views
- Groovy Server Pages (GSP) or JSON Views - Responsible for the "V" in MVC. -
commands
- Custom Grails Commands - Create your own Grails CLI commands.
-
-
src/main/groovy
- Supporting Sources -
src/test/groovy
- Unit Tests -
src/integration-test/groovy
- Integration Tests - For testing Grails applications at the integration level. -
src/main/scripts
- Code generation scripts.
Understanding this directory structure and its conventions is fundamental to efficient Grails development.
2.8 Running and Debugging an Application
Grails applications can be run with the built in Tomcat server using the run-app command which will load a server on port 8080 by default:
grails run-app
You can specify a different port by using the -port
argument:
grails run-app -port=8090
Note that it is better to start up the application in interactive mode since a container restart is much quicker:
$ grails
grails> run-app
| Grails application running at http://localhost:8080 in environment: development
grails> stop-app
| Shutting down application...
| Application shutdown.
grails> run-app
| Grails application running at http://localhost:8080 in environment: development
You can debug a grails app by simply right-clicking on the Application.groovy
class in your IDE and choosing the appropriate action (since Grails 3).
Alternatively, you can run your app with the following command and then attach a remote debugger to it.
grails run-app --debug-jvm
More information on the run-app command can be found in the reference guide.
Via Gradle, Grails applications can be executed using the built-in application server using the bootRun
command. By default, it launches a server on port 8080:
$ ./gradlew bootRun
To specify a different port, you can set the system property -Dgrails.server.port
as follows:
$ ./gradlew bootRun -Dgrails.server.port=8081
For debugging a Grails app, you have two options. You can either right-click on the Application.groovy
class in your IDE and select the appropriate debugging action, or you can run the app with the following command and then connect a remote debugger to it:
$ ./gradlew bootRun --debug-jvm
For more information on the bootRun
command, please refer to the bootRun section of the Grails reference guide.
2.9 Testing an Application
The create-*
commands in Grails automatically create unit or integration tests for you within the src/test/groovy
directory. It is of course up to you to populate these tests with valid test logic, information on which can be found in the section on Unit and integration tests.
To execute tests you run the test-app command as follows:
grails test-app
Gradle offers a convenient feature where you can automatically generate unit and integration tests for your application using the create-*
commands. These generated tests are stored in the src/test/groovy
and src/integration-test/groovy
directories. However, it is your responsibility to populate these tests with the appropriate test logic. You can find comprehensive guidance on crafting valid test logic in the section dedicated to Unit and Integration Tests.
To initiate the execution of your tests, including both unit and integration tests, you can utilize the Gradle check
task. Follow these steps:
-
Open your terminal or command prompt and navigate to your Grails project’s root directory.
-
Execute the following Gradle command:
$ ./gradlew check
By running the
check
task, you ensure that all tests in your Grails project, including the ones you’ve created and populated with test logic, are executed. This comprehensive testing approach contributes significantly to the robustness and overall quality of your application. -
Viewing Test Reports: After running your tests, Grails generates test reports that provide valuable insights into the test results. You can typically find these reports in the
build/reports/tests
directory of your Grails project. Open these reports in a web browser to view detailed information about test outcomes, including passed, failed, and skipped tests.
Remember, testing is not just a process; it’s a fundamental practice that enhances your Grails application’s reliability. Viewing test reports helps you analyze and understand the test results, making it easier to identify and address any issues.
By following these testing practices and reviewing test reports, you can deliver a high-quality Grails application to your users with confidence.
2.10 Deploying an Application
Grails applications can be deployed in a number of different ways.
If you are deploying to a traditional container (Tomcat, Jetty etc.) you can create a Web Application Archive (WAR file), and Grails includes the war command for performing this task:
grails war
This will produce a WAR file under the build/libs
directory which can then be deployed as per your container’s instructions.
Note that by default Grails will include an embeddable version of Tomcat inside the WAR file, this can cause problems if you deploy to a different version of Tomcat. If you don’t intend to use the embedded container then you should change the scope of the Tomcat dependencies to testImplementation
(or provided
if using Grails 4.x) prior to deploying to your production container in build.gradle
:
testImplementation "org.springframework.boot:spring-boot-starter-tomcat"
If you are building a WAR file to deploy on Tomcat 7 then in addition you will need to change the target Tomcat version in the build. Grails is built against Tomcat 8 APIs by default.
To target a Tomcat 7 container, insert a line to build.gradle
above the dependencies { }
section:
ext['tomcat.version'] = '7.0.59'
Grails 5 contains dependencies that require javax.el-api:3.0 (eg.: datastore-gorm:7.x , spring-boot:2.x ) which is only supported starting from Tomcat 8.x+, based on the tomcat version table!
|
Unlike most scripts which default to the development
environment unless overridden, the war
command runs in the production
environment by default. You can override this like any script by specifying the environment name, for example:
grails dev war
If you prefer not to operate a separate Servlet container then you can simply run the Grails WAR file as a regular Java application. Example:
grails war
java -Dgrails.env=prod -jar build/libs/mywar-0.1.war
When deploying Grails you should always run your containers JVM with the -server
option and with sufficient memory allocation. A good set of VM flags would be:
-server -Xmx768M
Via Gradle, Grails applications offer multiple deployment options.
For traditional container deployments, such as Tomcat or Jetty, you can generate a Web Application Archive (WAR) file using the Gradle war
task as follows:
$ ./gradlew war
This task generates a WAR file with a -plain
suffix within the build/libs
directory, ready for deployment according to your container’s guidelines.
By default, the war
task runs in the production
environment. You can specify a different environment, such as development
, by overriding it in the Gradle command:
$ ./gradlew -Dgrails.env=dev war
If you prefer not to use a separate Servlet container, you can create and run the Grails WAR file as a regular Java application:
$ ./gradlew bootWar
$ java -jar build/libs/mywar-0.1.war
When deploying Grails, ensure that your container’s JVM runs with the -server
option and sufficient memory allocation. Here are recommended VM flags:
-server -Xmx1024M
2.11 Supported Jakarta EE Containers
The Grails framework requires that runtime containers support Servlet 6.0.0 and above. By default, Grails framework applications are bundled with an embedded Tomcat server. For more information, please see the Deployment section of this documentation.
In addition, read the Grails Guides for tips on how to deploy Grails to various popular Cloud services.
2.12 Creating Artefacts
Grails provides a set of useful CLI commands for various tasks, including the creation of essential artifacts such as controllers and domain classes. These commands simplify the development process, although you can achieve similar results using your preferred Integrated Development Environment (IDE) or text editor.
For instance, to create the foundation of an application, you typically need to generate a domain model using Grails Commands:
The create-app
and create-domain-class
commands are supported in both the Grails Shell CLI and the Grails Forge CLI.
Grails Forge CLI
$ grails -t forge create-app myapp
$ cd myapp
$ grails -t forge create-domain-class book
Grails Shell CLI
$ grails create-app myapp
$ cd myapp
$ grails create-domain-class book
Executing these commands will result in the creation of a domain class located at grails-app/domain/myapp/Book.groovy
, as shown in the following code:
package myapp
class Book {
}
The Grails CLI offers numerous other commands that you can explore in the Grails command line reference guide.
Using interactive mode enhances the development experience by providing auto-complete and making the process smoother. |
2.13 Generating an Application
Quick Start with Grails Scaffolding
To get started quickly with Grails it is often useful to use a feature called scaffolding to generate the skeleton of an application. To do this use one of the generate-*
commands such as generate-all, which will generate a controller (and its unit test) and the associated views:
grails generate-all helloworld.Book
Gradle Quick Start with Grails Scaffolding
To quickly initiate your Grails project, you can employ the runCommand
Gradle task. This task allows you to generate the essential structure of an application swiftly. Specifically, when running the following command, you can create a controller (including its unit tests) and the associated views for your application:
$ ./gradlew runCommand -Pargs="generate-all myapp.Book"